Python API Code Example
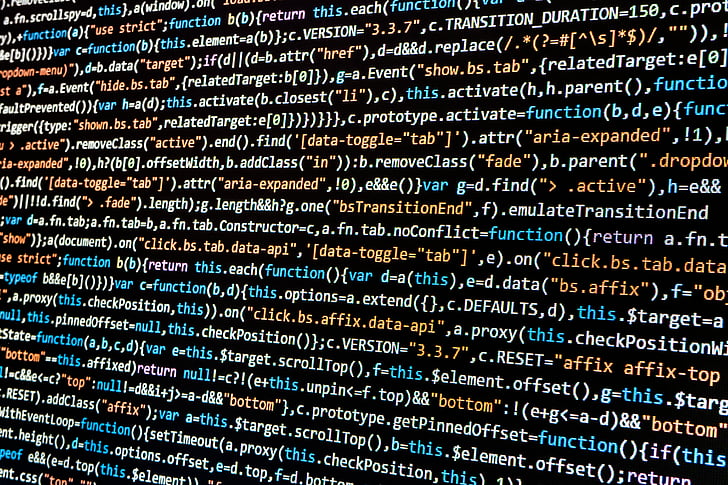
Getting API access with Python Code:
APIs, or application programming interfaces, have been transforming the way we interact with software and exchange information for years. By allowing different applications to communicate with each other and exchange data, APIs have opened up a world of possibilities for businesses, developers, and consumers alike.
In this article, we'll explore what APIs are, how they work, and why they're becoming increasingly important in today's connected world.
What is an API?
An API is a set of protocols, routines, and tools for building software and applications. It specifies how software components should interact. APIs allow for communication between two separate software systems. A software system implementing an API contains functions or sub-routines that can be executed by another software system.
APIs are the building blocks that enable developers to create new applications by leveraging existing services, data, and functionality. For example, if a business wants to create a new mobile app that displays weather data, it could use an API provided by a weather service to retrieve the data, and display it within the app.
APIs are designed to be easy to use, enabling developers to quickly integrate new features into their applications. This means that businesses can rapidly bring new products and services to market.
How do APIs work?
APIs work by using a request-response model, where a requesting system sends a request for data or a request to perform a specific action. The API returns the requested information or performs the requested action.
The requesting system (known as the client) sends a request to the API (known as the server) using a specific protocol, such as HTTP. The API processes the request, retrieves the necessary data, and sends a response back to the client. This response contains the requested information or confirmation that the requested action was completed.
APIs use a variety of standards and protocols, such as REST and SOAP, to ensure that the request and response are formatted correctly and can be processed by the client and server.
Why are APIs important?
APIs are becoming increasingly important for a number of reasons:
- Connecting systems: APIs allow different systems to communicate and exchange information. This enables new applications and services to be built by leveraging existing functionality.
- Driving innovation: APIs provide a way for businesses to quickly and easily integrate new features and functionality into their applications. This helps drive innovation, and enables new products and services to be brought to market more quickly.
- Enhancing user experiences: By allowing different applications to communicate and exchange data, APIs make it possible to create new and improved user experiences.
- Improving efficiency: APIs can automate repetitive tasks and improve the efficiency of business processes by allowing different systems to work together and share information.
- Enabling business growth: APIs provide businesses with new revenue streams by allowing them to monetize their data and services. This enables them to quickly and easily expand into new markets and reach new customers.
import requests
#access random user api and put in response variable
response = requests.get("https://randomuser.me/api/")
#look at header of api
response.headers
#find out what kind of content you are dealing with, in this case it is JSON
response.headers.get("content-type")
#look at the JSON content
response.json()
#create new file called randomperson and write content to it
#file = open("randomperson", "wb")
#file.write(response.content)
#file.close()
{'results': [{'gender': 'female', 'name': {'title': 'Mrs', 'first': 'محیا', 'last': 'محمدخان'}, 'location': {'street': {'number': 7924, 'name': 'یادگار امام'}, 'city': 'خوی', 'state': 'یزد', 'country': 'Iran', 'postcode': 98024, 'coordinates': {'latitude': '13.6071', 'longitude': '137.9115'}, 'timezone': {'offset': '-9:00', 'description': 'Alaska'}}, 'email': '[email protected]', 'login': {'uuid': 'a981cf6f-f0c8-4c93-bbdb-45ca9cf2d711', 'username': 'sadfrog422', 'password': 'soccer12', 'salt': 'cVIXJmBS', 'md5': '4a02d158ac25a5fe76cc4581677d1557', 'sha1': '6f1d8fd57cd63ebc69c561738b40b59b08dd6c2a', 'sha256': '264fb9a45cb340c241a6fa7c3a43a6f7046473c5ef48a7af2cb9d9fabd37132c'}, 'dob': {'date': '1990-02-07T18:04:04.269Z', 'age': 32}, 'registered': {'date': '2015-04-23T02:58:19.278Z', 'age': 7}, 'phone': '079-18410891', 'cell': '0971-860-5298', 'id': {'name': '', 'value': None}, 'picture': {'large': 'https://randomuser.me/api/portraits/women/59.jpg', 'medium': 'https://randomuser.me/api/portraits/med/women/59.jpg', 'thumbnail': 'https://randomuser.me/api/portraits/thumb/women/59.jpg'}, 'nat': 'IR'}], 'info': {'seed': '21fd97f5b7e29bbc', 'results': 1, 'page': 1, 'version': '1.4'}}
Interested in learning more Python code? Visit our friends at: CODE ACADEMY
Check out some information about Search Engine Optimization here: SEO Albany NY